- SCAN: In SCAN algorithm the disk arm moves into a particular direction and services the requests coming in its path and after reaching the end of disk, it reverses its direction and again services the request arriving in its path. So, this algorithm works as an elevator and hence also known as elevator algorithm.
- In SCAN disk scheduling algorithm, head starts from one end of the disk and moves towards the other end, servicing requests in between one by one and reach the other end. Then the direction of the head is reversed and the process continues as head continuously scan back and forth to access the disk.
- Disk-Scheduling solver Danger alert This front-end is obsolute! Use: nicomedes.assistedcoding.eu instead!!! Click here for Instructions Other problems About.
- OS Disk Scheduling Algorithms implementations in C and JAVA scheduling scan operating-system disk-scheduling fcfs sstf look disk-scheduling-algorithms Updated Sep 30, 2020.
- Sstf Disk Algorithm Coding Pdf
- Sstf Disk Scheduling Algorithm Program In Java
- Sstf Disk Scheduling Algorithm Program In C
- Sstf Disk Algorithm Coding Questions
Disk Scheduling Algorithms
Algorithm of the SSTF is given below:- Find the positive distance of all tracks in the request array from the head. Find a track from the requested array which has not been accessed/serviced yet and has a minimum distance from the head. Increment the total seek count with this distance.
In a multi-programming, when the disk controller is busy servicing a request from a process, any new requests from other process are placed in a queue. When the disk controller finishes the current request it has to decide which request from the queue should be processed next. How does the OS make this choice? It can choose one of the many disk scheduling algorithms to make the decision. Some of the common disk scheduling algorithms are:
- First Come First Serve (FCFS)
- Shortest Seek Time First (SSTF)
- SCAN
- LOOK
First Come First Serve (FCFS)
In FCFS the disk controller chooses the next request in the queue to service.
e.g. On a disk with 200 cylinders (0-199), find the number of cylinders the disk arm must move considering a disk queue with requests for I/O to blocks on cylinders
98, 183, 37, 122, 14, 124, 65, 67
Assume the current Head pointer (position) is 53rd cylinder
Ans:
The order of request serviced will be:
53 -> 98 -> 183 -> 37 -> 122 -> 14 -> 124 -> 65 -> 67
Thus, the total head movement is |98-53|+|183-98|+|37-183|+|122-37|+|14-122|+124-14|+|65-124|+|67-65| = 45+85+146+85+108+110+59+2=640
Shortest Seek Time First (SSTF)
SSTF selects the request with the least seek time from the current head position. SSTF chooses the pending request closest to the current head position.
e.g. for the same question (as in FCFS), the order of request serviced using SSTF will be:
53 -> 65 -> 67 -> 37 -> 14 -> 98 -> 122 -> 124 -> 183
Thus, the total head movement is |65-53|+|67-65|+|37-67|+|14-37|+|98-14|+|122-98|+|124-122|+|183-124| = 12+2+30+23+84+24+2+59 = 236
SCAN
The disk arm starts at one end of the disk and moves towards other end, servicing requests as it reaches each cylinder. At the other end, the direction of head movement is reversed, and servicing continues.
e.g. for the same question (as in FCFS), the order of request serviced using SCAN will be:
53 -> 37 -> 14 -> 0 -> 65 -> 67 -> 98 -> 122 -> 124 -> 183
Thus, the total head movement is |37-53|+|14-37|+|0-14|+|65-0|+|67-65|+|98-67|+|122-98|+|124-122|+|183-124| = 16+23+14+65+2+31+24+2+59=236
LOOK
Same as SCAN but with the difference that the arm goes only as far as the final request in each direction. Then, it reverses direction immediately without going all the way to the end of the disk.
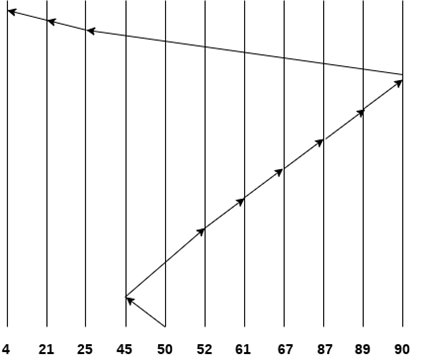
e.g. for the same question (as in FCFS), the order of request serviced using LOOK will be:
53 -> 37 -> 14 -> 65 -> 67 -> 98 -> 122 -> 124 -> 183
Thus, the total head movement is |37-53|+|14-37|+|65-14|+|67-65|+|98-67|+|122-98|+|124-122|+|183-124| = 16+23+51+2+31+24+2+59=208
PPT
The above discussion is summarized in the form of a PPT with animations below.
Note: Click within the slide area for animations. Clicking on the “next” slide button will not display any animation
Let us learn how to implement shortest seek time first algorithm in C programming with its explanation, output, advantages, disadvantages and much more.
What is Shortest Seek Time First Disk Scheduling Algorithm?
The shortest seek time first is commonly abbreviated as SSTF. It is also popularly known as shortest seek first algorithm.
The SSTF disk scheduling algorithm is a secondary storage scheduling algorithm which enables to determine the motion of the disk’s arm and the disk head in servicing read and write requests.
Sstf Disk Algorithm Coding Pdf
This algorithm helps to determine which job is nearest to the current head position with minimum seek time, and then services that job next.
In the SSTF algorithm, the jobs having the shortest seek time are executed first. Therefore, the seek time of each job is pre-calculated in the job queue and every job is scheduled according to its seek time.
Sstf Disk Scheduling Algorithm Program In Java
This enables the job nearest to the disk arm to get executed first. It also has a better average response time and throughput as compared to the FCFS scheduling algorithm.
Advantages
- Reduced total seek time as compared to FCFS algorithm
- Increased throughput time
- Decreased average response time
Disadvantages
- There are high chances of starvation if the seek time is higher than the incoming jobs
- Switching the directions may slow down the process
- There are chances of overheads.
- This algorithm is not the most optimal one
Note: This C program to implement SSTF algorithm is compiled with GNU GCC compiler using CodeLite IDE on Microsoft Windows 10 operating system.
C Program For Shortest Seek Time First Disk Scheduling Algorithm
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70 72 74 76 | { intflag; { intarray_1[33],array_2[33]; intcount=0,j,x,limit,minimum,location,disk_head,sum=0; scanf('%d',&limit); scanf('%d',&disk_head); while(count<limit) scanf('%d',&h[count].num); count++; for(count=0;count<limit;count++) x=0; location=0; { { { if(array_1[j]<0) array_1[j]=h[j].num-disk_head; minimum=array_1[j]; x++; else array_1[j]=disk_head-h[j].num; { } if(minimum>array_1[j]) minimum=array_1[j]; } } array_2[count]=h[location].num-disk_head; { } } while(count<limit) sum=sum+array_2[count]; } printf('nTotal movements of the cylinders:t%d',sum); } |
Output
Sstf Disk Scheduling Algorithm Program In C
If you have any doubts about the implementation of shortest seek first disk scheduling C program, let us know about it in the comment section. Find more about it here.
Sstf Disk Algorithm Coding Questions
CPU Scheduling Algorithms |
---|
FCFS Algorithm |
Preemptive Shortest Job First Algorithm |
Round Robin Algorithm |
Shortest Job First Algorithm |
C SCAN Scheduling C Program |
SCAN Algorithm |
Multi-Level Feedback Queue Algorithm |
Preemptive Priority Algorithm |
Priority Scheduling Algorithm |